Hall Sensor Tutorial for Arduino, ESP8266 and ESP32
In this tutorial we cover the Hall Sensor, a sensor to measure magnetic fields.
First we describe what the Hall Effect is and how a hall effect can be measured from a sensor.
After we know the fundamentals, we cover two different sensors:
- Linear Hall Sensor
- Magnetic Hall Sensor
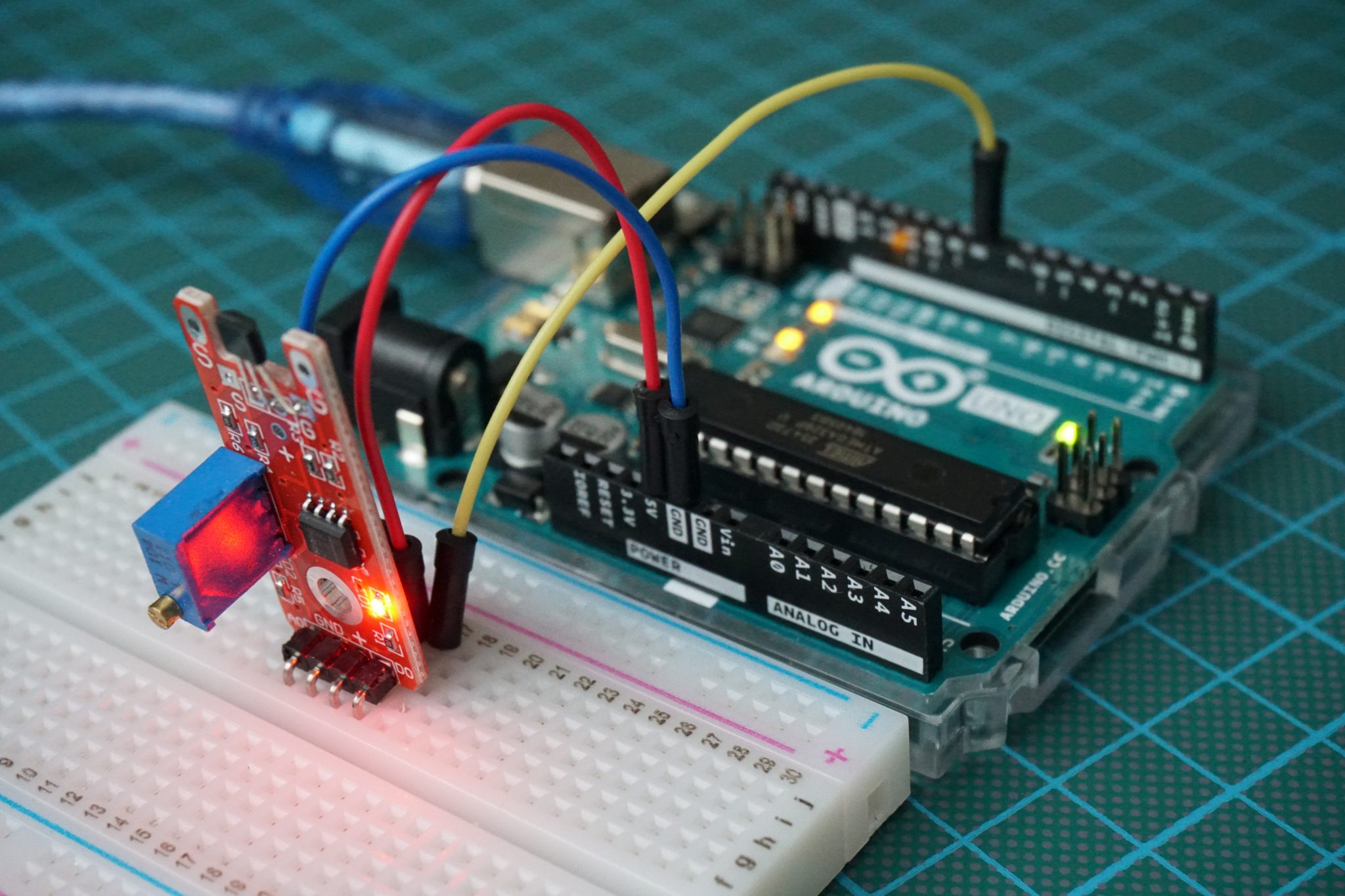
Table of Contents
What is the Hall Effect?
If electric current flows through a conductor, a magnetic field is employed perpendicular to the current direction. This magnetic field exerts a diagonal force on the moving charge carriers which tends to push them to one side of the conductor. Because there is a potential difference due to the pushed outwards charge carries a measurable voltage between the two sides of the conductor is produced, the so called Hall voltage. This effect is called the Hall Effect after E. H. Hall who discovered it in 1879. The effect is depending on the thickness of the conductor. Therefore the conductor should be very thin.
From the picture we see how a hall sensor is working. We need a power supply (5V and GND) and also a second circuit to measure the Hall voltage (input of the microcontroller).
Typical applications of Hall sensors are:
- Brushless DC electric motors to detect the position of the permanent magnet.
- Computer printers to detect missing paper and open covers.
- General switch applications because of the following advantages:
- Lower costs of production compared to a mechanical switch.
- Higher reliability compared to a mechanical switch.
- Higher operational frequencies than a mechanical switch.
- No debouncing effect (see button and switch article)
- Hall sensor as a switch can be completely build in a case and is therefore protected against dirt and water.
One disadvantage of a Hall Effect sensor is the much lower measuring accuracy compared to fluxgate magnetometers or magnetoresistance-based sensor. But these sensors are quite more expensive for our DIY projects.
The following table gives you an overview of all components and parts that I used for this tutorial. I get commissions for purchases made through links in this table.
Arduino Uno | Amazon | Banggood | AliExpress | |
OR | ESP8266 NodeMCU | Amazon | Banggood | AliExpress |
OR | ESP32 NodeMCU | Amazon | Banggood | AliExpress |
AND | Linear Hall Sensor (KY-024) and Magnetic Hall Sensor (KY-003) in Sensor Pack | Amazon | Banggood | AliExpress |
Linear Hall Sensor (KY-024)
The KY-024 Linear magnetic Hall sensor reacts in the presence of a magnetic field. It has a build in potentiometer on the module to adjust the sensitivity of the sensor and it provides both analog and digital outputs. The operating voltage of the KY-024 linear hall sensor is between 2.7V and 6.5V and therefore suitable for Arduino (operating voltage: 5V) and ESP (operating voltage: 3.3V) microcontroller.
- The digital output acts as a switch that will turn on/off when a magnet is near, similar to the KY-003.
- Digital outout = 1: no magnetic field
- Digital outout = 0: magnetic field
- The analog output can measure the polarity and relative strength of the magnetic field.
The following pictures show the wiring between the KY-024 linear hall sensor and the Arduino, ESP8266 or ESP32 microcontroller.
After the wiring we can create two Arduino script:
- Reads the analog value of the hall sensor and print the values to the serial plotter of the Arduino IDE to show the strength and polarity of the magnetic field.
- Read the digital value of the KY-024 and print the measurement to the serial monitor to show the status of the switch.
You do not have to change the wiring between the two examples because the connection between hall sensor and microcontroller is available, but we only include the connection in the program code that we need.
Linear Hall Sensor Analog Output
The following script shows how to read the analog sensor values of the linear hall sensor and print them on the serial connection to view the values in the Serial plotter of the Arduino IDE.
int analogPin = A0; // for Arduino microcontroller
//int analogPin = A0; // for ESP8266 microcontroller
//int analogPin = 4; // for ESP32 microcontroller
void setup() {
pinMode(analogPin, INPUT);
Serial.begin(9600);
}
void loop() {
int analogVal = analogRead(analogPin);
Serial.println(analogVal);
delay(100);
}
Because this script can be used for Arduino, EPS8266 and ESP32 microcontroller boards, you only need one of the first three lines where we define the analog pin of the connection. You can delete or comment the two lines that you do not need.
After we defined the pin that is the analog input for the microcontroller, we have to define this pin as input pin in the setup function. Also we set the baud rate of the serial communication to 9600, that has to be the same in the Arduino IDE to plot the sensor values.
In the loop function, we read the analog values with the analogRead function and save the analog value to a variable. Then we print the value to the serial monitor and use a short delay of 0.1 seconds before we start the loop function again to read the next analog sensor value.
The following video shows the strength of the magnetic field and also the polarity with the spikes up and down.
Linear Hall Sensor Digital Output
As second example we want to measure the digital value of the linear hall sensor. Because the signal is digital and only gives us a 0 or 1, we do not know the strength of the magnetic field, but know that our threshold, calibrated with the potentiomenter, was exceeded if we get the 0 as result of the measurement.
We have to make sure that the sensitivity of the sensor is well calibrated with the potentiometer. Therefore if your digital sensor values are not changing and your wiring setup is correct, you can either use a stronger magnet or turn the potentiometer, while the sensor is near the magnet, until the digital values are changing.
int digitalPin = 7; // for Arduino microcontroller
//int digitalPin = D7; // for ESP8266 microcontroller
//int digitalPin = 0; // for ESP32 microcontroller
void setup() {
pinMode(digitalPin, INPUT);
Serial.begin(9600);
}
void loop() {
int digitalVal = digitalRead(digitalPin);
Serial.println(digitalVal);
delay(100);
}
The program code does not differ much from the previous example, where we read the analog values. In the first part of the script, we change the pins to the digital connection and choose the microcontroller that we use.
The setup function is completely the same and in the loop function we change the name of the variable that holds the digital value and also change the function from analogRead to digitalRead the with previous defined digitalPin.
The following videos shows how I change the polarity of the magnet and also the distance between the magnet and the KY-024 linear hall sensor. The picture shows a screenshot of the serial monitor of the Arduino IDE, where the digital values changes between 0 and 1.
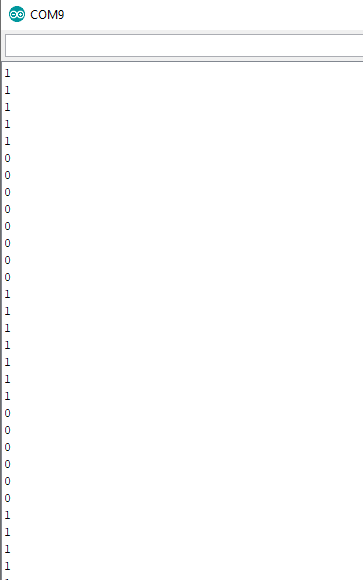
Magnetic Hall Sensor (KY-003)
The KY-003 magnetic Hall sensor is a digital sensor and is therefore only able to operate as switch. Maybe you know a reed switch? I also wrote an entire article about this switch, you can find here. The reed switch is also a magnetic switch. But how do you know if you better use a magnetic hall sensor as switch or a reed switch? In the following table I compared the two different magnetic switches with the advantages and disadvantages.
Magnetic Hall Sensor (KY-003) | Reed Switch (KY-025, KY-021) | |
Switch Function | Transducer that varies the output voltage depending on the presents of a magnetic field. | Pair of ferrous metal contacts. If contracts are open, there is no electrical contact. The contacts are closed by a magnet near the switch and opened by removing the magnet. |
Magnet – Sensor Orientation | Magnet has to be perpendicular to magnetic hall sensor | Magnet has to be parallel to reed switch |
Advantages |
|
|
Disadvantages |
|
|
It is not possible to measure how strong the magnetic field is with the magnetic hall sensor. The operation voltage of the KY-003 is between 3.3V and 5V. This module is able to operate under high temperature conditions due to the maximal operating temperature of 85°C or 358 Kelvin.
In the following example we want to switch the magnetic hall sensor on and off. But you can not turn off or on the sensor with the same polarity. Therefore I have to change the polarity to trigger the magnetic sensor, which you also see in the video at the end of this article. To visualize if the sensor or the switch is turned on or off, I use a LED and the digital signal of the KY-003.
The following pictures show the wiring between the KY-003 magnetic hall sensor and the Arduino, ESP8266 or ESP32 microcontroller.
For the program code we need to make a couple of changes because we want to turn the LED on and off based on the current status of the magnet hall sensor.
// for Arduino microcontroller
int led = 9;
int hallsensor = 8;
// for ESP8266 microcontroller
//int led = D7;
//int hallsensor = D8;
// for ESP32 microcontroller
//int led = 0;
//int hallsensor = 4;
void setup() {
pinMode(led, OUTPUT);
pinMode(hallsensor, INPUT);
}
void loop() {
int digitalVal = digitalRead(hallsensor);
if (digitalVal == LOW) {
digitalWrite (led, HIGH);
}
else {
digitalWrite (led, LOW);
}
delay(100);
}
At the beginning of the script we have to define on which digital I/O pins are the LED and the hall sensor connected. Because this script is for Arduino, ESP8266 and ESP32 microcontrollers, you have to comment the desired sections or delete the lines for the microcontroller that you do not need.
In the setup function, we define the LED pin as output and the pin of the hall sensor as input.
The loop function start with reading the digital value from the KY-003 hall sensor. If the digital state is 0 (equal to LOW) we turn on the LED because there is a magnetic field recognized. Otherwise we turn the LED off. At the end of the script we include a short delay of 0.1 seconds before we start the loop function again.
The following video shows that the LED is turned on, when the magnet comes near the hall sensor. But if the magnet is not present, the status of the hall sensor does not change. The digital value is still 0 and therefore the LED is on. Only when the polarity of the magnet is changed, we can reset the magnetic hall sensor. The digital value returns to 1 and the LED goes off.
Conclusion
Hall sensors are a nice way to realize projects, where the sensor itself is hidden. An example could be that you want to update a system or restart a system, where the whole system is build in a wall. In this case you could trigger the action with a simple magnet touching the wall.
Did you liked the article? If you have any questions, please use the comment section below to ask.
Hi! I was wondering what exactly the unit is that’s recorded by the Linear Hall Sensor – the one that equates 5V to 1023?
Yes, the onboard Analog to Digital converter converts the voltage to a digital value between 0 and 1023 where 5V is equivalent to 1023.
Can the Hall sensor output (5V) be connected to the input of an ESP (3.3V)?
Hi Gabriel, yes the Hall sensor can be operated with 5V as well as 3.3V
Just to clarify, you can operate the depicted linear Hall sensor module with 3.3 or 5 Volts. But DO NOT feed a 5V-powered Hall sensor output into a 3.3V logic. You would need a logic level converter in between the two.
Thank you very much for this tutorial! I tried it myself with the KY-024 Hall sensor. Here my result: https://nerd-corner.com/hallsensor-ky-024-arduino-code/